Spring MVC + jQuery Autocomplete example
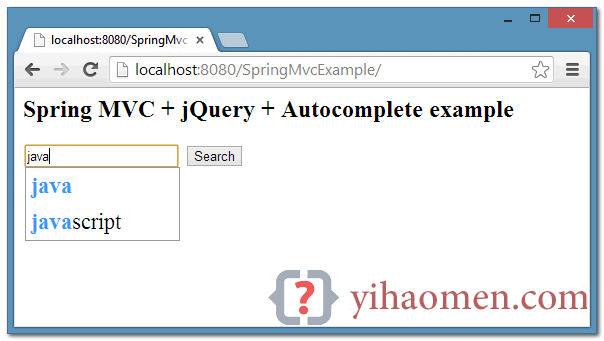
In this tutorial, we show you how to integrate jQuery autocomplete plugin with Spring MVC.
Tools and Libraries used :
- Spring MVC 3.2
- jQuery autocomplete plugin – github
- Maven 3
- Eclipse IDE
Review the tutorial’s flows :
- An HTML page, with a textbox.
- If the user is tying on the textbox, it will fire an Ajax request (via autocomplete plugin) to Spring controller.
- Spring process the user input and return the search result (in JSON format).
- The “autocomplete plugin” process the returned result and display the autocomplete suggestion box. See figure above.
1. Project Directory
Review the final project directory structure, a standard Maven project.
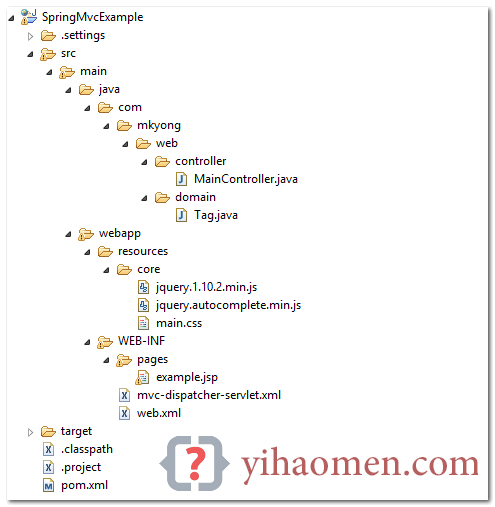
2. Project Dependencies
Declares Spring, JSTL and Jackson(JSON result).
<properties> <spring.version>3.2.2.RELEASE</spring.version> <jstl.version>1.2</jstl.version> <jackson.version>1.9.10</jackson.version> </properties> <dependencies> <!-- jstl --> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> <version>${jstl.version}</version> </dependency> <!-- Spring Core --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <!-- need this for @Configuration --> <dependency> <groupId>cglib</groupId> <artifactId>cglib</artifactId> <version>2.2.2</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <!-- Jackson --> <dependency> <groupId>org.codehaus.jackson</groupId> <artifactId>jackson-mapper-asl</artifactId> <version>${jackson.version}</version> </dependency> </dependencies>
3. Spring MVC – Data Provider
A Spring controller, if user issue a “/getTags” request, Spring will filter out the result with user input and return it in JSON format.
package com.mkyong.web.controller; import java.util.ArrayList; import java.util.List; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.servlet.ModelAndView; import com.mkyong.web.domain.Tag; @Controller public class MainController { List<Tag> data = new ArrayList<Tag>(); MainController() { // init data for testing data.add(new Tag(1, "ruby")); data.add(new Tag(2, "rails")); data.add(new Tag(3, "c / c++")); data.add(new Tag(4, ".net")); data.add(new Tag(5, "python")); data.add(new Tag(6, "java")); data.add(new Tag(7, "javascript")); data.add(new Tag(8, "jscript")); @RequestMapping(value = "/", method = RequestMethod.GET) public ModelAndView getPages() { ModelAndView model = new ModelAndView("example"); return model; @RequestMapping(value = "/getTags", method = RequestMethod.GET) public @ResponseBody List<Tag> getTags(@RequestParam String tagName) { return simulateSearchResult(tagName); private List<Tag> simulateSearchResult(String tagName) { List<Tag> result = new ArrayList<Tag>(); // iterate a list and filter by tagName for (Tag tag : data) { if (tag.getTagName().contains(tagName)) { result.add(tag); return result;
package com.mkyong.web.domain; public class Tag { public int id; public String tagName; //getter and setter methods public Tag(int id, String tagName) { this.id = id; this.tagName = tagName;
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <context:component-scan base-package="com.mkyong" /> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix"> <value>/WEB-INF/pages/</value> </property> <property name="suffix"> <value>.jsp</value> </property> </bean> <mvc:resources mapping="/resources/**" location="/resources/" /> <mvc:annotation-driven /> </beans>
4. jQuery Automplete Plugin
The JSP page below should be self-explanatory.
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%> <html> <head> <script src="<c:url value="/resources/core/jquery.1.10.2.min.js" />"></script> <script src="<c:url value="/resources/core/jquery.autocomplete.min.js" />"></script> <link href="<c:url value="/resources/core/main.css" />" rel="stylesheet"> </head> <body> <h2>Spring MVC + jQuery + Autocomplete example</h2> <div> <input type="text" id="w-input-search" value=""> <span> <button id="button-id" type="button">Search</button> </span> </div> <script> $(document).ready(function() { $('#w-input-search').autocomplete({ serviceUrl: '${pageContext.request.contextPath}/getTags', paramName: "tagName", delimiter: ",", transformResult: function(response) { return { //must convert json to javascript object before process suggestions: $.map($.parseJSON(response), function(item) { return { value: item.tagName, data: item.id }; }) }; }); }); </script> </body> </html>
#1. The autocomplete plugin will generate the suggestions list with following HTML tags.
<div class="autocomplete-suggestions"> <div class="autocomplete-suggestion autocomplete-selected">...</div> <div class="autocomplete-suggestion">...</div> <div class="autocomplete-suggestion">...</div> </div>
So, you need to style it, for example :
.autocomplete-suggestions { border: 1px solid #999; background: #FFF; overflow: auto; } .autocomplete-suggestion { padding: 5px 5px; white-space: nowrap; overflow: hidden; font-size:22px} .autocomplete-selected { background: #F0F0F0; } .autocomplete-suggestions strong { font-weight: bold; color: #3399FF; }
#2. Fro this “autocomplete plugin”, the response from the server must be a JSON formatted JavaScript object like this :
suggestions: [ { value: "Java", data: "1001" }, { value: "JavaScript", data: "1002" }, { value: "Ruby", data: "1003" }
#3. Review the following Ajax request
$('#w-input-search').autocomplete({ serviceUrl: '${pageContext.request.contextPath}/getTags', paramName: "tagName", // ?tagName='user input' delimiter: ",", transformResult: function(response) { return { suggestions: $.map($.parseJSON(response), function(item) { return { value: item.tagName, data: item.id }; }) }; } });
- serviceUrl – Server side URL or callback function that returns the JSON data.
- paramName – In this case, it will generate getTags?tagName=user input. Default is ?query=user input.
- delimiter – For multiple suggestions.
- $.parseJSON (response) – Java will return JSON formatted data, so, you need to convert it to a JavaScript object.
5. Demo
Start and access “http://localhost:8080/SpringMvcExample/“.
Type “java”
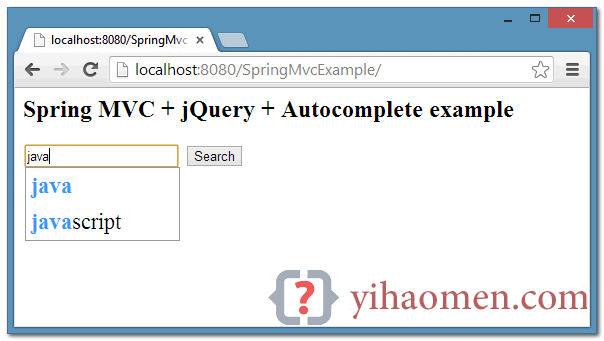
Type “r”
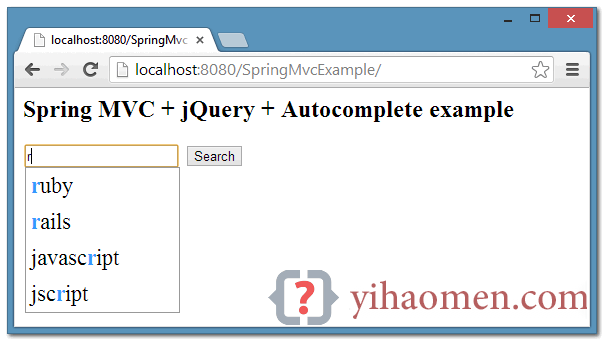
References
- jQuery autocomplete plugin
- Create a Simple Autocomplete With HTML5 & jQuery
- 35+ Best Ajax jQuery Autocomplete Tutorial & Plugin with Examples
- jQuery $.parseJSON example
- jQuery $.map() example
- jQuery $.each() example
- Wikipedia : JSON
From:一号门
COMMENTS