TestNG + Selenium Load Testing Example
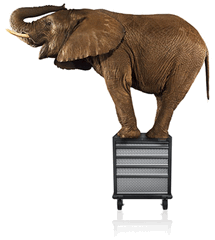
In this tutorial, we will show you how to use @Test attributes invocationCount and threadPoolSize to perform a load test or stress test on a website.
Tools used :
- TestNG 6.8.7
- Selenium 2.39.0
- Maven 3
P.S We are using the Selenium library to automate browsers to access a website.
1. Project Dependency
Get TestNG and Selenium libraries.
<properties> <testng.version>6.8.7</testng.version> <selenium.version>2.39.0</selenium.version> </properties> <dependencies> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>${testng.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>${selenium.version}</version> </dependency> <dependencies>
2. @Test(invocationCount=?)
This invocationCount determined how many times TestNG should run this test method.
Example 2.1
@Test(invocationCount = 10) public void repeatThis() { //...
Output – The repeatThis() method will run 10 times.
PASSED: repeatThis PASSED: repeatThis PASSED: repeatThis PASSED: repeatThis PASSED: repeatThis PASSED: repeatThis PASSED: repeatThis PASSED: repeatThis PASSED: repeatThis PASSED: repeatThis
Example 2.2 – Uses Selenium to open a Firefox browser and load “Google.com”. This test is to make sure the page title is always “Google”.
package com.mkyong.testng.examples.loadtest; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.Assert; import org.testng.annotations.Test; public class TestMultipleThreads { @Test(invocationCount = 5) public void loadTestThisWebsite() { WebDriver driver = new FirefoxDriver(); driver.get("http://www.google.com"); System.out.println("Page Title is " + driver.getTitle()); Assert.assertEquals("Google", driver.getTitle()); driver.quit();
Output – You will notice that Firefox browser will prompts out and close 5 times.
Page Title is Google Page Title is Google Page Title is Google Page Title is Google Page Title is Google PASSED: loadTestThisWebsite PASSED: loadTestThisWebsite PASSED: loadTestThisWebsite PASSED: loadTestThisWebsite PASSED: loadTestThisWebsite
3. @Test(invocationCount = ?, threadPoolSize = ?)
The threadPoolSize attribute tells TestNG to create a thread pool to run the test method via multiple threads. With thread pool, it will greatly decrease the running time of the test method.
Example 3.1 – Start a thread pool, which contains 3 threads, and run the test method 3 times.
@Test(invocationCount = 3, threadPoolSize = 3) public void testThreadPools() { System.out.printf("Thread Id : %s%n", Thread.currentThread().getId());
Output – The test method run 3 times, and each received its own thread.
[ThreadUtil] Starting executor timeOut:0ms workers:3 threadPoolSize:3 Thread Id : 10 Thread Id : 12 Thread Id : 11 PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools
Example 3.2 – Start a thread pool, which contains 3 threads, and run the test method 10 times.
@Test(invocationCount = 10, threadPoolSize = 3) public void testThreadPools() { System.out.printf("Thread Id : %s%n", Thread.currentThread().getId());
Output – The test method run 10 times, and the threads are reused.
[ThreadUtil] Starting executor timeOut:0ms workers:10 threadPoolSize:3 Thread Id : 10 Thread Id : 11 Thread Id : 12 Thread Id : 10 Thread Id : 11 Thread Id : 12 Thread Id : 10 Thread Id : 11 Thread Id : 12 Thread Id : 10 PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools PASSED: testThreadPools
4. Load Test Example
By combining both TestNG multiple threads and Selenium powerful browser automation. You can create a simple yet powerful load test like this :
package com.mkyong.testng.examples.loadtest; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.Assert; import org.testng.annotations.Test; public class TestMultipleThreads { @Test(invocationCount = 100, threadPoolSize = 5) public void loadTest() { System.out.printf("%n[START] Thread Id : %s is started!", Thread.currentThread().getId()); WebDriver driver = new FirefoxDriver(); driver.get("http://yourwebsite.com"); //perform whatever actions, like login, submit form or navigation System.out.printf("%n[END] Thread Id : %s", Thread.currentThread().getId()); driver.quit();
Output – Above test will start a thread pool of 5, and send 100 URL requests to a specified website.
[ThreadUtil] Starting executor timeOut:0ms workers:100 threadPoolSize:5 [START] Thread Id : 11 is started! [START] Thread Id : 14 is started! [START] Thread Id : 10 is started! [START] Thread Id : 12 is started! [START] Thread Id : 13 is started! [END] Thread Id : 11 [START] Thread Id : 11 is started! [END] Thread Id : 10 [START] Thread Id : 10 is started! [END] Thread Id : 13 [START] Thread Id : 13 is started! [END] Thread Id : 14 [START] Thread Id : 14 is started! [END] Thread Id : 12 [START] Thread Id : 12 is started! [END] Thread Id : 13 ......
Q : For load testing with Selenium and TestNG, why only one browser is prompts out at a time?
A : Your test method is completed too fast, try putting a Thread.sleep(5000) to delay the execution, now, you should notice multiple browsers prompt out simultaneously. (For demonstration purpose only).
References
From:一号门
Previous:Donate to Charity
COMMENTS