Ant and jUnit Task example
By:Roy.LiuLast updated:2019-08-17
In this tutorial, we will show you how to run a junit test in Ant build.
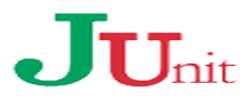
1. Run a unit test
build.xml
<target name="junit" depends="compile"> <junit printsummary="yes" haltonfailure="no"> <!-- Project classpath, must include junit.jar --> <classpath refid="test.path" /> <!-- test class --> <classpath location="${test.classes.dir}" /> <test name="com.mkyong.test.TestMessage" haltonfailure="no" todir="${report.dir}"> <formatter type="plain" /> <formatter type="xml" /> </test> </junit> </target>
2. Run a batch of unit test
build.xml
<target name="junit" depends="compile"> <junit printsummary="yes" haltonfailure="no"> <!-- <classpath location="lib/junit-4.11.jar" /> <classpath location="lib/hamcrest-core-1.3.jar" /> --> <classpath refid="test.path" /> <classpath location="${test.classes.dir}" /> <formatter type="xml" /> <formatter type="plain" /> <batchtest fork="yes" todir="${report.dir}"> <fileset dir="${test.dir}"> <include name="**/*Test*.java" /> </fileset> </batchtest> </junit> </target>
3. Example
A web application example to show you how to run a junit test.
3.1 Return a message
MessageGenerator.java
package com.mkyong.message; import org.springframework.stereotype.Component; @Component public class MessageGenerator { public String getWelcomeMessage() { return "welcome";
3.2 Two junit test cases to test above class.
TestMessage.java
package com.mkyong.test; import static org.junit.Assert.assertEquals; import org.junit.Test; import com.mkyong.message.MessageGenerator; public class TestMessage { @Test public void test_welcome_message() { MessageGenerator obj = new MessageGenerator(); assertEquals("welcome", obj.getWelcomeMessage());
TestMessage2.java
package com.mkyong.test; import static org.junit.Assert.assertEquals; import org.junit.Test; import com.mkyong.message.MessageGenerator; public class TestMessage2 { @Test public void test_welcome_message_2() { MessageGenerator obj = new MessageGenerator(); assertEquals("welcome", obj.getWelcomeMessage());
3.3 Use ivy to get the project dependencies, and declared the project scope.
ivy.xml
<ivy-module version="2.0"> <info organisation="org.apache" module="WebProject" /> <configurations> <conf name="compile" description="Required to compile application"/> <conf name="runtime" description="Additional run-time dependencies" extends="compile"/> <conf name="test" description="Required for test only" extends="runtime"/> </configurations> <dependencies> <dependency org="junit" name="junit" rev="4.11" conf="test->default" /> </dependencies> </ivy-module>
3.4 Run unit test
build.xml
<project xmlns:ivy="antlib:org.apache.ivy.ant" name="HelloProject" default="main" basedir="."> <description> Running junit Test </description> <!-- Project Structure --> <property name="jdk.version" value="1.7" /> <property name="projectName" value="WebProject" /> <property name="src.dir" location="src" /> <property name="test.dir" location="src" /> <property name="report.dir" location="report" /> <property name="web.dir" value="war" /> <property name="web.classes.dir" location="${web.dir}/WEB-INF/classes" /> <!-- ivy start --> <target name="resolve" description="retrieve dependencies with ivy"> <echo message="Getting dependencies..." /> <ivy:retrieve /> <ivy:cachepath pathid="compile.path" conf="compile" /> <ivy:cachepath pathid="runtime.path" conf="runtime" /> <ivy:cachepath pathid="test.path" conf="test" /> </target> <!-- Compile Java source from ${src.dir} and output it to ${web.classes.dir} --> <target name="compile" depends="init, resolve" description="compile source code"> <mkdir dir="${web.classes.dir}" /> <javac destdir="${web.classes.dir}" source="${jdk.version}" target="${jdk.version}" debug="true" includeantruntime="false" classpathref="compile.path"> <src path="${src.dir}" /> </javac> </target> <!-- Run jUnit --> <target name="junit" depends="compile"> <junit printsummary="yes" haltonfailure="no"> <classpath refid="test.path" /> <classpath location="${web.classes.dir}" /> <formatter type="xml" /> <batchtest fork="yes" todir="${report.dir}"> <fileset dir="${test.dir}"> <include name="**/*Test*.java" /> </fileset> </batchtest> </junit> </target> <!-- Create folders --> <target name="init"> <mkdir dir="${src.dir}" /> <mkdir dir="${web.classes.dir}" /> <mkdir dir="${report.dir}" /> </target> <!-- Delete folders --> <target name="clean" description="clean up"> <delete dir="${web.classes.dir}" /> <delete dir="${report.dir}" /> </target> <target name="main" depends="junit" /> </project> Run it <pre><code class="language-bash"> $ ant junit
Done.
References
From:一号门
Previous:Maven Get source code for Jar
COMMENTS