Java Custom Exception Examples
By:Roy.LiuLast updated:2019-08-17
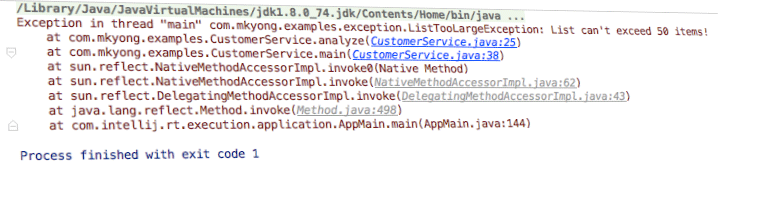
In Java, there are two types of exceptions – checked and unchecked exception. Here’s the summary :
- Checked – Extends java.lang.Exception, for recoverable condition, try-catch the exception explicitly, compile error.
- Unchecked – Extends java.lang.RuntimeException, for unrecoverable condition, like programming errors, no need try-catch, runtime error.
1. Custom Checked Exception
Note
Some popular checked exception : IOException, FileNotFoundException
Some popular checked exception : IOException, FileNotFoundException
1.1 If the client is able to recover from the exception, make it a checked exception. To create a custom checked exception, extends java.lang.Exception
NameNotFoundException.java
package com.mkyong.examples.exception; public class NameNotFoundException extends Exception { public NameNotFoundException(String message) { super(message);
1.2 For checked exception, you need to try and catch the exception.
CustomerService.java
package com.mkyong.examples; import com.mkyong.examples.exception.NameNotFoundException; public class CustomerService { public Customer findByName(String name) throws NameNotFoundException { if ("".equals(name)) { throw new NameNotFoundException("Name is empty!"); return new Customer(name); public static void main(String[] args) { CustomerService obj = new CustomerService(); try { Customer cus = obj.findByName(""); } catch (NameNotFoundException e) { e.printStackTrace();
Output
com.mkyong.examples.exception.NameNotFoundException: Name is empty! at com.mkyong.examples.CustomerService.findByName(CustomerService.java:10) at com.mkyong.examples.CustomerService.main(CustomerService.java:39) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:144)
2. Custom Unchecked Exception
Note
Some popular unchecked exception : NullPointerException, IndexOutOfBoundsException, IllegalArgumentException
Some popular unchecked exception : NullPointerException, IndexOutOfBoundsException, IllegalArgumentException
2.1 If the client cannot do anything to recover from the exception, make it an unchecked exception. To create a custom unchecked exception, extends java.lang.RuntimeException
ListTooLargeException.java
package com.mkyong.examples.exception; public class ListTooLargeException extends RuntimeException{ public ListTooLargeException(String message) { super(message);
2.3 For unchecked exception, try and catch the exception is optional.
CustomerService.java
package com.mkyong.examples; import com.mkyong.examples.exception.ListTooLargeException; import java.util.ArrayList; import java.util.Collections; import java.util.List; public class CustomerService { public void analyze(List<String> data) { if (data.size() > 50) { //runtime exception throw new ListTooLargeException("List can't exceed 50 items!"); //... public static void main(String[] args) { CustomerService obj = new CustomerService(); //create 100 size List<String> data = new ArrayList<>(Collections.nCopies(100, "mkyong")); obj.analyze(data);
Output
Exception in thread "main" com.mkyong.examples.exception.ListTooLargeException: List can't exceed 50 items! at com.mkyong.examples.CustomerService.analyze(CustomerService.java:25) at com.mkyong.examples.CustomerService.main(CustomerService.java:38) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:144)
References
- Unchecked Exceptions — The Controversy
- difference between java.lang.RuntimeException and java.lang.Exception
From:一号门
COMMENTS