Spring Boot file upload example
This article shows you how to upload a file in Spring Boot web application.
Tools used :
- Spring Boot 1.4.3.RELEASE
- Spring 4.3.5.RELEASE
- Thymeleaf
- Maven
- Embedded Tomcat 8.5.6
1. Project Structure
A standard project structure.
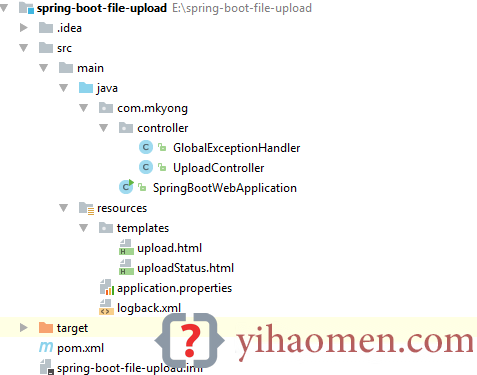
2. Project Dependency
Spring boot dependencies, no need extra library for file upload.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mkyong</groupId> <artifactId>spring-boot-file-upload</artifactId> <packaging>jar</packaging> <version>1.0</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.4.3.RELEASE</version> </parent> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <!-- hot swapping, disable cache for template, enable live reload --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency> </dependencies> <build> <plugins> <!-- Package as an executable jar/war --> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
3. File Upload Example
Spring boot file upload, zero configuration.
3.1 In the Controller, maps the uploaded file to MultipartFile
package com.mkyong.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.multipart.MultipartFile; import org.springframework.web.servlet.mvc.support.RedirectAttributes; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; @Controller public class UploadController { //Save the uploaded file to this folder private static String UPLOADED_FOLDER = "F://temp//"; @GetMapping("/") public String index() { return "upload"; @PostMapping("/upload") // //new annotation since 4.3 public String singleFileUpload(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) { if (file.isEmpty()) { redirectAttributes.addFlashAttribute("message", "Please select a file to upload"); return "redirect:uploadStatus"; try { // Get the file and save it somewhere byte[] bytes = file.getBytes(); Path path = Paths.get(UPLOADED_FOLDER + file.getOriginalFilename()); Files.write(path, bytes); redirectAttributes.addFlashAttribute("message", "You successfully uploaded '" + file.getOriginalFilename() + "'"); } catch (IOException e) { e.printStackTrace(); return "redirect:/uploadStatus"; @GetMapping("/uploadStatus") public String uploadStatus() { return "uploadStatus";
3.2 In thymeleaf, just some normal HTML file tags.
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <body> <h1>Spring Boot file upload example</h1> <form method="POST" action="/upload" enctype="multipart/form-data"> <input type="file" name="file" /><br/><br/> <input type="submit" value="Submit" /> </form> </body> </html>
3.3 Another page for upload status
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <body> <h1>Spring Boot - Upload Status</h1> <div th:if="${message}"> <h2 th:text="${message}"/> </div> </body> </html>
4. Max upload size exceeded
To handle the max upload size exceeded exception, declares a @ControllerAdvice and catch the MultipartException
package com.mkyong.controller; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.multipart.MultipartException; import org.springframework.web.servlet.mvc.support.RedirectAttributes; @ControllerAdvice public class GlobalExceptionHandler { //https://jira.spring.io/browse/SPR-14651 //Spring 4.3.5 supports RedirectAttributes @ExceptionHandler(MultipartException.class) public String handleError1(MultipartException e, RedirectAttributes redirectAttributes) { redirectAttributes.addFlashAttribute("message", e.getCause().getMessage()); return "redirect:/uploadStatus"; /* Spring < 4.3.5 @ExceptionHandler(MultipartException.class) public String handleError2(MultipartException e) { return "redirect:/errorPage"; }*/
5. Tomcat Connection Reset
If you deployed to Tomcat, configure the maxSwallowSize to avoid this Tomcat connection reset issue. For embedded Tomcat, declares a TomcatEmbeddedServletContainerFactory like the following :
package com.mkyong; import org.apache.coyote.http11.AbstractHttp11Protocol; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.context.embedded.tomcat.TomcatConnectorCustomizer; import org.springframework.boot.context.embedded.tomcat.TomcatEmbeddedServletContainerFactory; import org.springframework.context.annotation.Bean; @SpringBootApplication public class SpringBootWebApplication { private int maxUploadSizeInMb = 10 * 1024 * 1024; // 10 MB public static void main(String[] args) throws Exception { SpringApplication.run(SpringBootWebApplication.class, args); //Tomcat large file upload connection reset //http://www.mkyong.com/spring/spring-file-upload-and-connection-reset-issue/ @Bean public TomcatEmbeddedServletContainerFactory tomcatEmbedded() { TomcatEmbeddedServletContainerFactory tomcat = new TomcatEmbeddedServletContainerFactory(); tomcat.addConnectorCustomizers((TomcatConnectorCustomizer) connector -> { if ((connector.getProtocolHandler() instanceof AbstractHttp11Protocol<?>)) { //-1 means unlimited ((AbstractHttp11Protocol<?>) connector.getProtocolHandler()).setMaxSwallowSize(-1); }); return tomcat;
6. Multipart File Size
By default, Spring Boot max file upload size is 1MB, you can configure the values via following application properties :
#http://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/#common-application-properties #search multipart spring.http.multipart.max-file-size=10MB spring.http.multipart.max-request-size=10MB
7. DEMO
Start Spring Boot with the default embedded Tomcat mvn spring-boot:run.
$ mvn spring-boot:run . ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v1.4.3.RELEASE) 2017-01-21 07:48:53 INFO com.mkyong.SpringBootWebApplication - Starting SpringBootWebApplication on MKYONG-WIN10 with PID 2384 (E:\spring-boot-file-upload\target\classes started by mkyong in E:\spring-boot-file-upload) 2017-01-21 07:48:53 DEBUG com.mkyong.SpringBootWebApplication - Running with Spring Boot v1.4.3.RELEASE, Spring v4.3.5.RELEASE 2017-01-21 07:48:53 INFO com.mkyong.SpringBootWebApplication - No active profile set, falling back to default profiles: default 2017-01-21 07:48:55 INFO com.mkyong.SpringBootWebApplication - Started SpringBootWebApplication in 2.54 seconds (JVM running for 2.924)
7.1 Access http://localhost:8080/
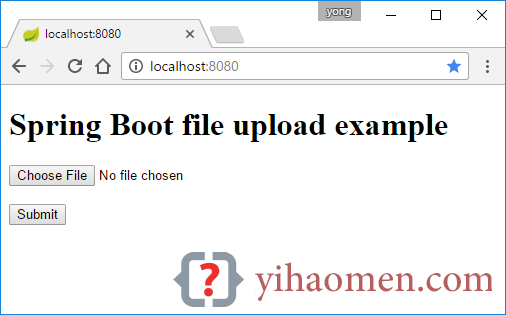
7.2 Select a file and upload it.
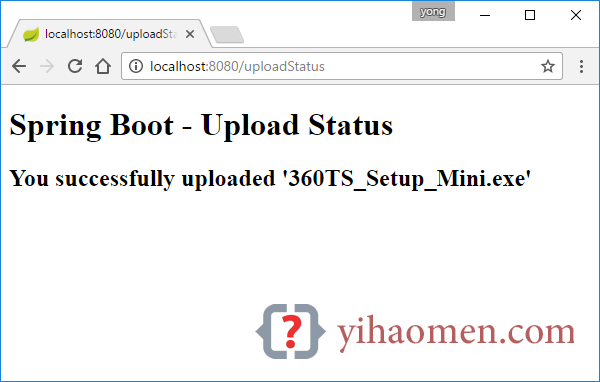
7.3 Select a file larger than 10mb, you will visit this page.
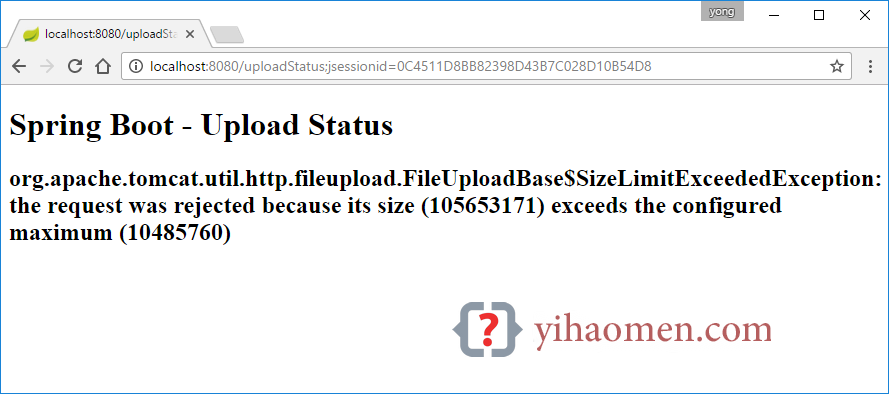
8. Download Source Code
References
- Spring Boot common application properties
- Spring MVC file upload example
- Spring @ExceptionHandler and RedirectAttributes
- Spring Boot Hello World Example – Thymeleaf
From:一号门
COMMENTS