Spring Boot non-web application example
By:Roy.LiuLast updated:2019-08-11
In Spring Boot, to create a non-web application, implements CommandLineRunner and override the run method, for example :
import org.springframework.boot.CommandLineRunner; @SpringBootApplication public class SpringBootConsoleApplication implements CommandLineRunner { public static void main(String[] args) throws Exception { SpringApplication.run(SpringBootConsoleApplication.class, args); //access command line arguments @Override public void run(String... args) throws Exception { //do something
1. Project Structure
A standard Maven project structure.
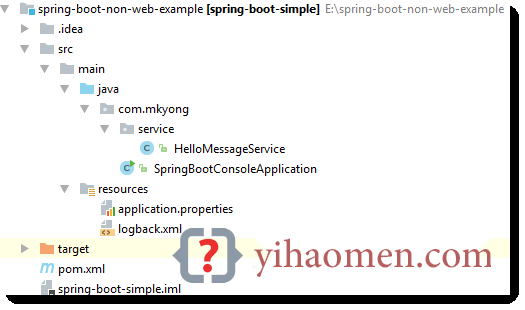
2. Project Dependency
Only spring-boot-starter
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mkyong</groupId> <artifactId>spring-boot-simple</artifactId> <packaging>jar</packaging> <version>1.0</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.1.RELEASE</version> </parent> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> </dependencies> <build> <plugins> <!-- Package as an executable jar/war --> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
3. Spring
3.1 A service to return a message.
HelloMessageService.java
package com.mkyong.service; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Service; @Service public class HelloMessageService { @Value("${name:unknown}") private String name; public String getMessage() { return getMessage(name); public String getMessage(String name) { return "Hello " + name;
application.properties
name=mkyong
3.2 CommandLineRunner example. If you run this Spring Boot, the run method will be the entry point.
SpringBootConsoleApplication.java
package com.mkyong; import com.mkyong.service.HelloMessageService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.Banner; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import static java.lang.System.exit; @SpringBootApplication public class SpringBootConsoleApplication implements CommandLineRunner { @Autowired private HelloMessageService helloService; public static void main(String[] args) throws Exception { //disabled banner, don't want to see the spring logo SpringApplication app = new SpringApplication(SpringBootConsoleApplication.class); app.setBannerMode(Banner.Mode.OFF); app.run(args); // Put your logic here. @Override public void run(String... args) throws Exception { if (args.length > 0) { System.out.println(helloService.getMessage(args[0].toString())); } else { System.out.println(helloService.getMessage()); exit(0);
4. DEMO
Package and run it.
## Go to project directory ## package it $ mvn package $ java -jar target/spring-boot-simple-1.0.jar Hello mkyong $ java -jar target/spring-boot-simple-1.0.jar "donald trump" Hello donald trump
References
From:一号门
COMMENTS