Spring Boot Profile based properties and yaml example
By:Roy.LiuLast updated:2019-08-11
In Spring Boot, it picks .properties or .yaml files in the following sequences :
- application-{profile}.properties and YAML variants
- application.properties and YAML variants
Note
For detail, sequence and order, please refer to this official Externalized Configuration documentation.
For detail, sequence and order, please refer to this official Externalized Configuration documentation.
Tested :
- Spring Boot 2.1.2.RELEASE
- Maven 3
1. Project Structure
A standard Maven project structure.
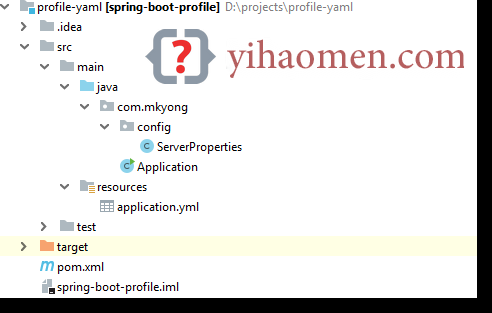
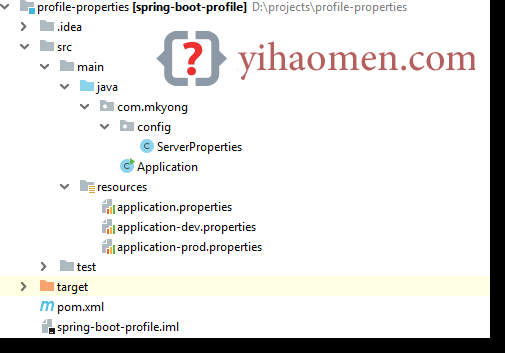
2. @ConfigurationProperties
Read the properties or yaml files later.
ServerProperties.java
package com.mkyong.config; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.stereotype.Component; import java.util.ArrayList; import java.util.List; @Component @ConfigurationProperties("server") public class ServerProperties { private String email; private List<Cluster> cluster = new ArrayList<>(); public static class Cluster { private String ip; private String path; public String getIp() { return ip; public void setIp(String ip) { this.ip = ip; public String getPath() { return path; public void setPath(String path) { this.path = path; @Override public String toString() { return "Cluster{" + "ip='" + ip + '\'' + ", path='" + path + '\'' + '}'; public String getEmail() { return email; public void setEmail(String email) { this.email = email; public List<Cluster> getCluster() { return cluster; public void setCluster(List<Cluster> cluster) { this.cluster = cluster; @Override public String toString() { return "ServerProperties{" + "email='" + email + '\'' + ", cluster=" + cluster + '}';
3. Profile based Properties
Multiple profiles .properties example.
application.properties
#Logging logging.level.org.springframework.web=ERROR logging.level.com.mkyong=ERROR logging.level.=error #spring spring.main.banner-mode=off spring.profiles.active=dev
application-dev.properties
#dev environment server.email: dev@mkyong.com server.cluster[0].ip=127.0.0.1 server.cluster[0].path=/dev1 server.cluster[1].ip=127.0.0.2 server.cluster[1].path=/dev2 server.cluster[2].ip=127.0.0.3 server.cluster[2].path=/dev3
application-prod.properties
#production environment server.email: prod@mkyong.com server.cluster[0].ip=192.168.0.1 server.cluster[0].path=/app1 server.cluster[1].ip=192.168.0.2 server.cluster[1].path=/app2 server.cluster[2].ip=192.168.0.3 server.cluster[2].path=/app3
4. Profile based YAML
Multiple profiles .yml example. In YAML, we can create multiple profiles by using a “—” separator.
application.yml
logging: level: .: error org.springframework: ERROR com.mkyong: ERROR spring: profiles: active: "dev" main: banner-mode: "off" server: email: default@mkyong.com --- spring: profiles: dev server: email: dev@mkyong.com cluster: - ip: 127.0.0.1 path: /dev1 - ip: 127.0.0.2 path: /dev2 - ip: 127.0.0.3 path: /dev3 --- spring: profiles: prod server: email: prod@mkyong.com cluster: - ip: 192.168.0.1 path: /app1 - ip: 192.168.0.2 path: /app2 - ip: 192.168.0.3 path: /app3
5. DEMO
5.1 Spring Boot application.
Application.java
package com.mkyong; import com.mkyong.config.ServerProperties; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class Application implements CommandLineRunner { @Autowired private ServerProperties serverProperties; @Override public void run(String... args) { System.out.println(serverProperties); public static void main(String[] args) { SpringApplication.run(Application.class, args);
5.2 Package and run it.
$ mvn package # The 'dev' profile is configured in application.properties # Profile : dev , picks application-dev.properties or YAML $ java -jar target/spring-boot-profile-1.0.jar ServerProperties{email='dev@mkyong.com', cluster=[ Cluster{ip='127.0.0.1', path='/dev1'}, Cluster{ip='127.0.0.2', path='/dev2'}, Cluster{ip='127.0.0.3', path='/dev3'} ]} # Profile : prod, picks application-prod.properties or YAML $ java -jar -Dspring.profiles.active=prod target/spring-boot-profile-1.0.jar ServerProperties{email='prod@mkyong.com', cluster=[ Cluster{ip='192.168.0.1', path='/app1'}, Cluster{ip='192.168.0.2', path='/app2'}, Cluster{ip='192.168.0.3', path='/app3'} ]} # Profile : abc, a non-exists profile $ java -jar -Dspring.profiles.active=abc target/spring-boot-profile-1.0.jar ServerProperties{email='null', cluster=[]}
Note
Spring Boot, the default profile is default, we can set the profile via spring.profiles.active property.
Spring Boot, the default profile is default, we can set the profile via spring.profiles.active property.
From:一号门
Previous:Spring Boot Tutorials
COMMENTS