JaCoCo Java Code Coverage + Maven example
Jacoco is used to measure the code coverage of application. In this tutorial we will understand how to configure Jacoco in maven and how to use Jacoco to see code coverage report.
Technologies Used:
- Eclipse Mars
- Maven 3.3.9
- Java 8
1. Eclipse Create Maven Java Project
1.1 In Eclipse create a Maven project File->New->Project->Maven Project, Select create a simple project and click on next
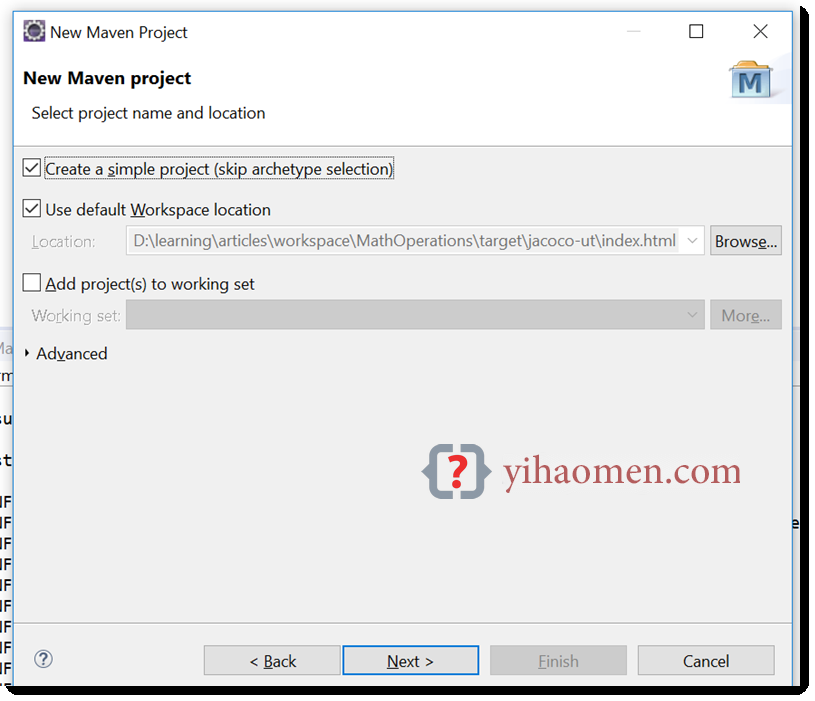
1.2 Enter groupId & artifactId as shown in below screen and click on finish.
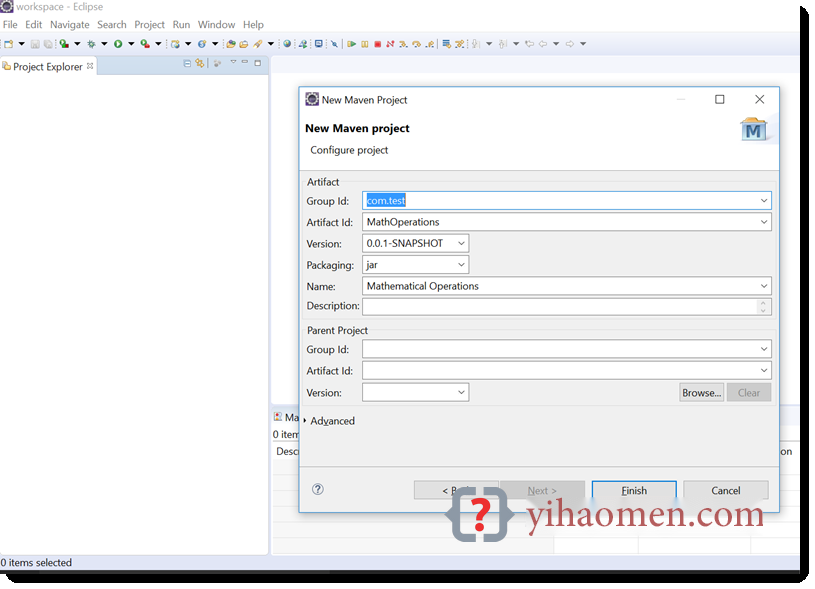
2. Project Structure
The project has the following components
- Sample Arithmetic Operations Class
- Sample Arithmetic Operations JUnit Test Class
- pom.xml with Junit and Jacoco dependencies
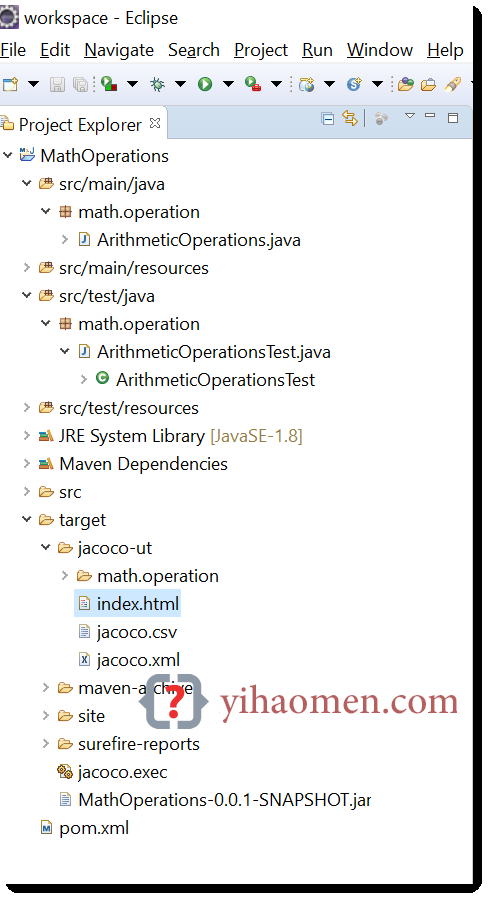
3. pom.xml
Configure Junit and Jacoco as shown in pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mkyong</groupId> <artifactId>MathOperations</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Mathematical Operations</name> <properties> <jacoco.version>0.7.5.201505241946</jacoco.version> <junit.version>4.12</junit.version> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.6.1</version> <configuration> <skipMain>true</skipMain> <skip>true</skip> <source>1.8</source> <target>1.8</target> </configuration> </plugin> <plugin> <groupId>org.jacoco</groupId> <artifactId>jacoco-maven-plugin</artifactId> <version>${jacoco.version}</version> <executions> <execution> <id>prepare-agent</id> <goals> <goal>prepare-agent</goal> </goals> </execution> <execution> <id>report</id> <phase>prepare-package</phase> <goals> <goal>report</goal> </goals> </execution> <execution> <id>post-unit-test</id> <phase>test</phase> <goals> <goal>report</goal> </goals> <configuration> <!-- Sets the path to the file which contains the execution data. --> <dataFile>target/jacoco.exec</dataFile> <!-- Sets the output directory for the code coverage report. --> <outputDirectory>target/jacoco-ut</outputDirectory> </configuration> </execution> </executions> <configuration> <systemPropertyVariables> <jacoco-agent.destfile>target/jacoco.exec</jacoco-agent.destfile> </systemPropertyVariables> </configuration> </plugin> </plugins> </build> </project>
4. Sample Arithmetic Operations Class
In this class method ‘add’ is created which accepts two integer parameters and returns sum.
package math.operation; public class ArithmeticOperations { public Integer add(Integer a, Integer b) return a+b;
5. Sample Arithmetic Operations Junit Test Class
Testcase is created for ‘add’ method.
package math.operation; import org.junit.Test; import static org.junit.Assert.assertEquals; public class ArithmeticOperationsTest { @Test public void testAdd() { ArithmeticOperations operations = new ArithmeticOperations(); Integer actual = operations.add(2, 6); Integer expected = 8; assertEquals(expected, actual);
6. Run Application
Right Click Project->Run as ->Maven test. Jacoco output report will be generated in target directory under jacoco-ut folder
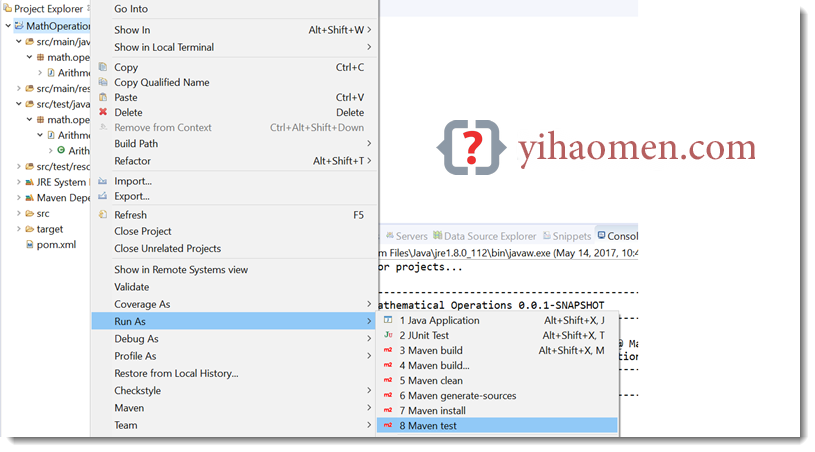
7. Output
7.1 To see the output go to target directory and open index.html from jacoco-ut folder in browser. Overall Report for class ArithmeticOperations is shown below
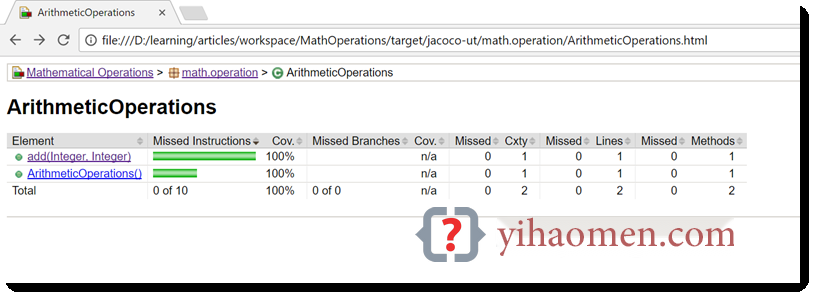
7.2 Clicking on each method in above figure gives detailed report. Here it shows green coloured line indicating which line is covered by unit test.
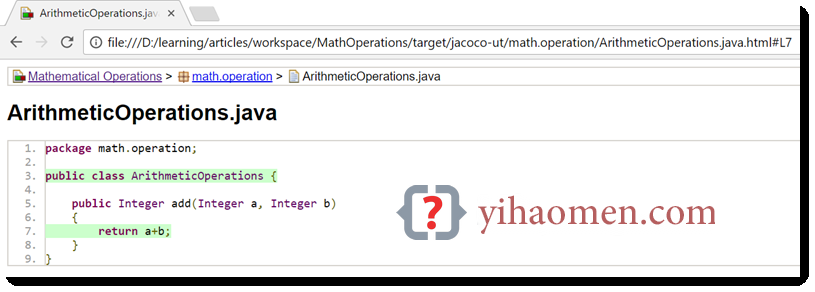
Done.
References
- JaCoCo Java Code Coverage Library
- Wikipedia – Java Code Coverage Tools
- JaCoCo – Maven Plug-in – EclEmma
From:一号门
COMMENTS