Spring Boot How to send email via SMTP
By:Roy.LiuLast updated:2019-08-11
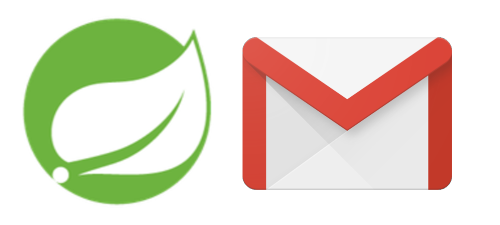
In this tutorial, we will show you how to send email via SMTP in Spring Boot.
Technologies used :
- Spring Boot 2.1.2.RELEASE
- Spring 5.1.4.RELEASE
- Java Mail 1.6.2
- Maven 3
- Java 8
1. Project Directory
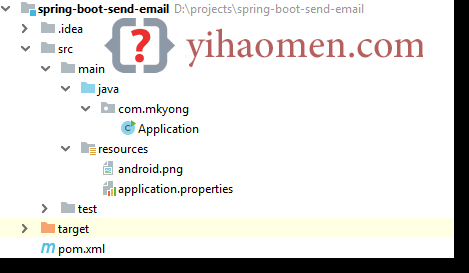
2. Maven
To send email, declares spring-boot-starter-mail, it will pull the JavaMail dependencies.
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <artifactId>spring-boot-send-email</artifactId> <packaging>jar</packaging> <name>Spring Boot Send Email</name> <url>https://www.mkyong.com</url> <version>1.0</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.2.RELEASE</version> </parent> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <!-- send email --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency> </dependencies> <build> <plugins> <!-- Package as an executable jar/war --> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.22.0</version> </plugin> </plugins> </build> </project>
Display the project dependencies.
$ mvn dependency:tree [INFO] org.springframework.boot:spring-boot-send-email:jar:1.0 [INFO] +- org.springframework.boot:spring-boot-starter:jar:2.1.2.RELEASE:compile [INFO] | +- org.springframework.boot:spring-boot:jar:2.1.2.RELEASE:compile [INFO] | | \- org.springframework:spring-context:jar:5.1.4.RELEASE:compile [INFO] | | +- org.springframework:spring-aop:jar:5.1.4.RELEASE:compile [INFO] | | \- org.springframework:spring-expression:jar:5.1.4.RELEASE:compile [INFO] | +- org.springframework.boot:spring-boot-autoconfigure:jar:2.1.2.RELEASE:compile [INFO] | +- org.springframework.boot:spring-boot-starter-logging:jar:2.1.2.RELEASE:compile [INFO] | | +- ch.qos.logback:logback-classic:jar:1.2.3:compile [INFO] | | | +- ch.qos.logback:logback-core:jar:1.2.3:compile [INFO] | | | \- org.slf4j:slf4j-api:jar:1.7.25:compile [INFO] | | +- org.apache.logging.log4j:log4j-to-slf4j:jar:2.11.1:compile [INFO] | | | \- org.apache.logging.log4j:log4j-api:jar:2.11.1:compile [INFO] | | \- org.slf4j:jul-to-slf4j:jar:1.7.25:compile [INFO] | +- javax.annotation:javax.annotation-api:jar:1.3.2:compile [INFO] | +- org.springframework:spring-core:jar:5.1.4.RELEASE:compile [INFO] | | \- org.springframework:spring-jcl:jar:5.1.4.RELEASE:compile [INFO] | \- org.yaml:snakeyaml:jar:1.23:runtime [INFO] \- org.springframework.boot:spring-boot-starter-mail:jar:2.1.2.RELEASE:compile [INFO] +- org.springframework:spring-context-support:jar:5.1.4.RELEASE:compile [INFO] | \- org.springframework:spring-beans:jar:5.1.4.RELEASE:compile [INFO] \- com.sun.mail:javax.mail:jar:1.6.2:compile [INFO] \- javax.activation:activation:jar:1.1:compile
3. Gmail SMTP
This example is using Gmail SMTP server, tested with TLS (port 587) and SSL (port 465). Read this Gmail SMTP
application.properties
spring.mail.host=smtp.gmail.com spring.mail.port=587 spring.mail.username=username spring.mail.password=password # Other properties spring.mail.properties.mail.smtp.auth=true spring.mail.properties.mail.smtp.connectiontimeout=5000 spring.mail.properties.mail.smtp.timeout=5000 spring.mail.properties.mail.smtp.writetimeout=5000 # TLS , port 587 spring.mail.properties.mail.smtp.starttls.enable=true # SSL, post 465 #spring.mail.properties.mail.smtp.socketFactory.port = 465 #spring.mail.properties.mail.smtp.socketFactory.class = javax.net.ssl.SSLSocketFactory
Note
For Gmail SMTP, if your account is enabled the 2-Step verification, please create an “App Password”. Read more on this JavaMail – Sending email via Gmail SMTP example
For Gmail SMTP, if your account is enabled the 2-Step verification, please create an “App Password”. Read more on this JavaMail – Sending email via Gmail SMTP example
4. Sending Email
Spring provides an JavaMailSender interface on top of JavaMail APIs.
4.1 Send a normal text email.
import org.springframework.mail.SimpleMailMessage; import org.springframework.mail.javamail.JavaMailSender; @Autowired private JavaMailSender javaMailSender; void sendEmail() { SimpleMailMessage msg = new SimpleMailMessage(); msg.setTo("to_1@gmail.com", "to_2@gmail.com", "to_3@yahoo.com"); msg.setSubject("Testing from Spring Boot"); msg.setText("Hello World \n Spring Boot Email"); javaMailSender.send(msg);
4.2 Send an HTML email and a file attachment.
import org.springframework.core.io.ClassPathResource; import org.springframework.mail.javamail.JavaMailSender; import org.springframework.mail.javamail.MimeMessageHelper; import javax.mail.MessagingException; import javax.mail.internet.MimeMessage; void sendEmailWithAttachment() throws MessagingException, IOException { MimeMessage msg = javaMailSender.createMimeMessage(); // true = multipart message MimeMessageHelper helper = new MimeMessageHelper(msg, true); helper.setTo("to_@email"); helper.setSubject("Testing from Spring Boot"); // default = text/plain //helper.setText("Check attachment for image!"); // true = text/html helper.setText("<h1>Check attachment for image!</h1>", true); // hard coded a file path //FileSystemResource file = new FileSystemResource(new File("path/android.png")); helper.addAttachment("my_photo.png", new ClassPathResource("android.png")); javaMailSender.send(msg);
5. Demo
5.1 Start Spring Boot, and it will send out an email.
Application.java
package com.mkyong; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.core.io.ClassPathResource; import org.springframework.mail.SimpleMailMessage; import org.springframework.mail.javamail.JavaMailSender; import org.springframework.mail.javamail.MimeMessageHelper; import javax.mail.MessagingException; import javax.mail.internet.MimeMessage; import java.io.IOException; @SpringBootApplication public class Application implements CommandLineRunner { @Autowired private JavaMailSender javaMailSender; public static void main(String[] args) { SpringApplication.run(Application.class, args); @Override public void run(String... args) { System.out.println("Sending Email..."); try { sendEmail(); //sendEmailWithAttachment(); } catch (MessagingException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); System.out.println("Done"); void sendEmail() { SimpleMailMessage msg = new SimpleMailMessage(); msg.setTo("1@gmail.com", "2@yahoo.com"); msg.setSubject("Testing from Spring Boot"); msg.setText("Hello World \n Spring Boot Email"); javaMailSender.send(msg); void sendEmailWithAttachment() throws MessagingException, IOException { MimeMessage msg = javaMailSender.createMimeMessage(); // true = multipart message MimeMessageHelper helper = new MimeMessageHelper(msg, true); helper.setTo("1@gmail.com"); helper.setSubject("Testing from Spring Boot"); // default = text/plain //helper.setText("Check attachment for image!"); // true = text/html helper.setText("<h1>Check attachment for image!</h1>", true); helper.addAttachment("my_photo.png", new ClassPathResource("android.png")); javaMailSender.send(msg);
Terminal
. ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v2.1.2.RELEASE) Sending Email... Done
From:一号门
COMMENTS