Java Whois example
By:Roy.LiuLast updated:2019-08-18
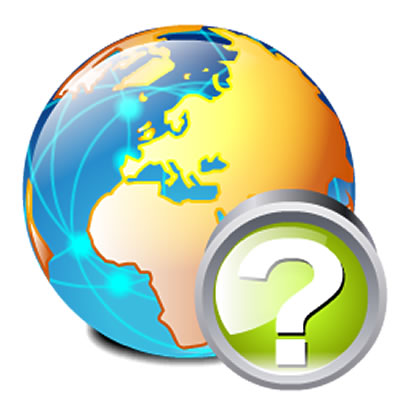
In this tutorial, we will show you how to use Java library – “Apache Commons Net” to get WHOIS data of a domain.
1. Simple Java Whois Example
For domain registered under internic.net, you can get the whois data directly.
WhoisTest.java
package com.mkyong.whois.bo; import java.io.IOException; import java.net.SocketException; import org.apache.commons.net.whois.WhoisClient; public class WhoisTest { public static void main(String[] args) { WhoisTest obj = new WhoisTest(); System.out.println(obj.getWhois("mkyong.com")); System.out.println("Done"); public String getWhois(String domainName) { StringBuilder result = new StringBuilder(""); WhoisClient whois = new WhoisClient(); try { //default is internic.net whois.connect(WhoisClient.DEFAULT_HOST); String whoisData1 = whois.query("=" + domainName); result.append(whoisData1); whois.disconnect(); } catch (SocketException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); return result.toString();
2. Complex Java Whois Example
For domain registered under other registrars (not internic.net), you need some extra steps to get the whois data.
Review the whois command in *nix and mac.
$ whois google.com ### It will returns many non related subdomain.google.com data $ whois =google.com ### Add '=' sign to get the exact result. ### refer to the "Whois server" URL ### Sample data ### Domain Name: GOOGLE.COM ### Registrar: MARKMONITOR INC. ### Whois Server: whois.markmonitor.com ### Referral URL: http://www.markmonitor.com ### The google.com whois is available under whois.markmonitor.com $ whois -h whois.markmonitor.com google.com ### done, finally, get the whois data of google.com
Let implement above steps in Java, see comments for self-explanatory.
WhoisTest.java
package com.mkyong.whois.bo; import java.io.IOException; import java.net.SocketException; import java.util.regex.Matcher; import java.util.regex.Pattern; import org.apache.commons.net.whois.WhoisClient; public class WhoisTest { private static Pattern pattern; private Matcher matcher; // regex whois parser private static final String WHOIS_SERVER_PATTERN = "Whois Server:\\s(.*)"; static { pattern = Pattern.compile(WHOIS_SERVER_PATTERN); public static void main(String[] args) { WhoisTest obj = new WhoisTest(); System.out.println(obj.getWhois("google.com")); System.out.println("Done"); // example google.com public String getWhois(String domainName) { StringBuilder result = new StringBuilder(""); WhoisClient whois = new WhoisClient(); try { whois.connect(WhoisClient.DEFAULT_HOST); // whois =google.com String whoisData1 = whois.query("=" + domainName); // append first result result.append(whoisData1); whois.disconnect(); // get the google.com whois server - whois.markmonitor.com String whoisServerUrl = getWhoisServer(whoisData1); if (!whoisServerUrl.equals("")) { // whois -h whois.markmonitor.com google.com String whoisData2 = queryWithWhoisServer(domainName, whoisServerUrl); // append 2nd result result.append(whoisData2); } catch (SocketException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); return result.toString(); private String queryWithWhoisServer(String domainName, String whoisServer) { String result = ""; WhoisClient whois = new WhoisClient(); try { whois.connect(whoisServer); result = whois.query(domainName); whois.disconnect(); } catch (SocketException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); return result; private String getWhoisServer(String whois) { String result = ""; matcher = pattern.matcher(whois); // get last whois server while (matcher.find()) { result = matcher.group(1); return result;
Output
Whois Server Version 2.0 Domain names in the .com and .net domains can now be registered with many different competing registrars. Go to http://www.internic.net for detailed information. //...... Registrant: Dns Admin Google Inc. Please contact contact-admin@google.com 1600 Amphitheatre Parkway Mountain View CA 94043 US dns-admin@google.com +1.6502530000 Fax: +1.6506188571 Domain Name: google.com Registrar Name: Markmonitor.com Registrar Whois: whois.markmonitor.com Registrar Homepage: http://www.markmonitor.com //...... Created on..............: 1997-09-15. Expires on..............: 2020-09-13. Record last updated on..: 2013-02-28. //......
Whois parser
Above is using a regex to grab the whois server url. If you know any Java whois parser, do comment below , thanks.
Above is using a regex to grab the whois server url. If you know any Java whois parser, do comment below , thanks.
References
From:一号门
COMMENTS