Spring MVC find location using IP Address (jQuery + Google Map)
By:Roy.LiuLast updated:2019-08-17
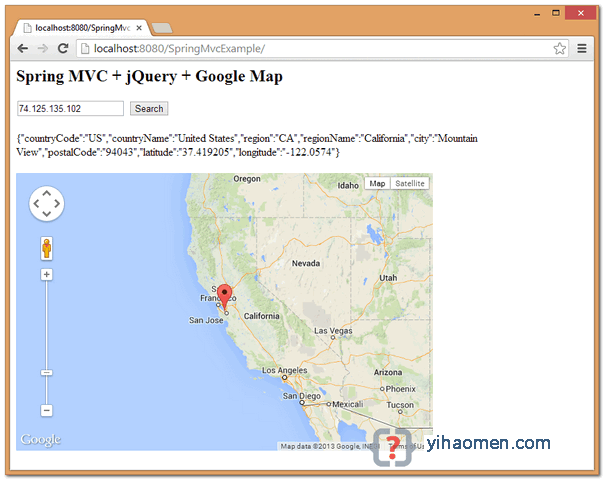
In this tutorial, we show you how to find a location using an IP address, with the following technologies :
- Spring MVC frameworks.
- jQuery (Ajax Request).
- GeoLite database.
- Google Map.
Review the tutorial’s flows
- A page with a text input and button.
- Type an IP address, and clicks on the button.
- jQuery fires an Ajax request to Spring Controller.
- Spring controller process and return back a json string.
- jQuery process the returned json and display the location on Google Map.
1. Project Directory
Review the final project directory structure, a standard Maven project.
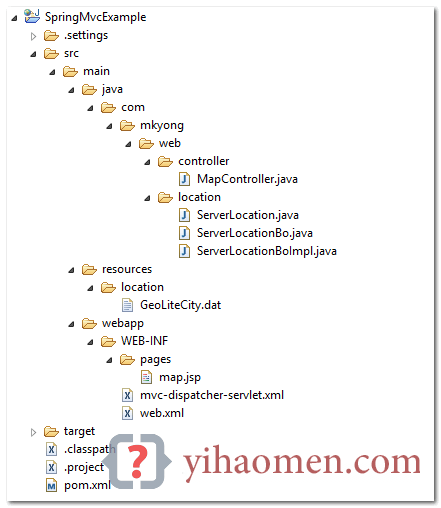
Note
Download the free GeoLite free Databases – GeoLiteCity.dat, and put it into the resources folder.
Download the free GeoLite free Databases – GeoLiteCity.dat, and put it into the resources folder.
2. Project Dependencies
Declares Spring frameworks, Jackson and geoip-api dependencies.
pom.xml
//... <properties> <spring.version>3.2.2.RELEASE</spring.version> <maxmind.geoip.version>1.2.10</maxmind.geoip.version> <jackson.version>1.9.10</jackson.version> </properties> <dependencies> <!-- Spring Core --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <!-- need this for @Configuration --> <dependency> <groupId>cglib</groupId> <artifactId>cglib</artifactId> <version>2.2.2</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <!-- ip to server location --> <dependency> <groupId>com.maxmind.geoip</groupId> <artifactId>geoip-api</artifactId> <version>${maxmind.geoip.version}</version> </dependency> <!-- Jackson --> <dependency> <groupId>org.codehaus.jackson</groupId> <artifactId>jackson-mapper-asl</artifactId> <version>${jackson.version}</version> </dependency> </dependencies> //...
3. Spring MVC + GeoLite
Get the location with GeoLite database.
ServerLocationBo.java
package com.mkyong.web.location; public interface ServerLocationBo { ServerLocation getLocation(String ipAddress);
ServerLocationBoImpl.java
package com.mkyong.web.location; import java.io.IOException; import java.net.URL; import org.springframework.stereotype.Component; import com.maxmind.geoip.Location; import com.maxmind.geoip.LookupService; import com.maxmind.geoip.regionName; @Component public class ServerLocationBoImpl implements ServerLocationBo { @Override public ServerLocation getLocation(String ipAddress) { String dataFile = "location/GeoLiteCity.dat"; return getLocation(ipAddress, dataFile); private ServerLocation getLocation(String ipAddress, String locationDataFile) { ServerLocation serverLocation = null; URL url = getClass().getClassLoader().getResource(locationDataFile); if (url == null) { System.err.println("location database is not found - " + locationDataFile); } else { try { serverLocation = new ServerLocation(); LookupService lookup = new LookupService(url.getPath(), LookupService.GEOIP_MEMORY_CACHE); Location locationServices = lookup.getLocation(ipAddress); serverLocation.setCountryCode(locationServices.countryCode); serverLocation.setCountryName(locationServices.countryName); serverLocation.setRegion(locationServices.region); serverLocation.setRegionName(regionName.regionNameByCode( locationServices.countryCode, locationServices.region)); serverLocation.setCity(locationServices.city); serverLocation.setPostalCode(locationServices.postalCode); serverLocation.setLatitude( String.valueOf(locationServices.latitude)); serverLocation.setLongitude( String.valueOf(locationServices.longitude)); } catch (IOException e) { System.err.println(e.getMessage()); return serverLocation;
ServerLocation.java
package com.mkyong.web.location; public class ServerLocation { private String countryCode; private String countryName; private String region; private String regionName; private String city; private String postalCode; private String latitude; private String longitude; //getter and setter methods
Spring controller, convert the ServerLocation with Jackson library, and return back a json string.
MapController.java
package com.mkyong.web.controller; import org.codehaus.jackson.map.ObjectMapper; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.servlet.ModelAndView; import com.mkyong.web.location.ServerLocation; import com.mkyong.web.location.ServerLocationBo; @Controller public class MapController { @Autowired ServerLocationBo serverLocationBo; @RequestMapping(value = "/", method = RequestMethod.GET) public ModelAndView getPages() { ModelAndView model = new ModelAndView("map"); return model; //return back json string @RequestMapping(value = "/getLocationByIpAddress", method = RequestMethod.GET) public @ResponseBody String getDomainInJsonFormat(@RequestParam String ipAddress) { ObjectMapper mapper = new ObjectMapper(); ServerLocation location = serverLocationBo.getLocation(ipAddress); String result = ""; try { result = mapper.writeValueAsString(location); } catch (Exception e) { e.printStackTrace(); return result;
4. JSP + jQuery + Google Map
When the search button is clicked, jQuery fire an Ajax request, process the data and update the Google Map.
map.jsp
<html> <head> <script src="http://maps.google.com/maps/api/js?sensor=true"></script> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> </head> <body> <h2>Spring MVC + jQuery + Google Map</h2> <div> <input type="text" placeholder="0.0.0.0" id="w-input-search" value=""> <span> <button id="w-button-search" type="button">Search</button> </span> </div> <script> $(document).ready(function() { $("#w-button-search").click(function() { $.getJSON("${pageContext.request.contextPath}/getLocationByIpAddress", ipAddress : $('#w-input-search').val() }, function(data) { var data = JSON.stringify(data); var json = JSON.parse(data); showMap(json["latitude"],json["longitude"]) $("#result").html(data) }) .done(function() { }) .fail(function() { }) .complete(function() { }); }); var map; function showMap(latitude,longitude) { var googleLatandLong = new google.maps.LatLng(latitude,longitude); var mapOptions = { zoom: 5, center: googleLatandLong, mapTypeId: google.maps.MapTypeId.ROADMAP }; var mapDiv = document.getElementById("map"); map = new google.maps.Map(mapDiv, mapOptions); var title = "Server Location"; addMarker(map, googleLatandLong, title, ""); function addMarker(map, latlong, title, content) { var markerOptions = { position: latlong, map: map, title: title, clickable: true }; var marker = new google.maps.Marker(markerOptions); }); </script> <br/> <div id="result"></div> <br/> <div style="width:600px;height:400px" id="map"></div> </body> </html>
5. Demo
IP Address : 74.125.135.102
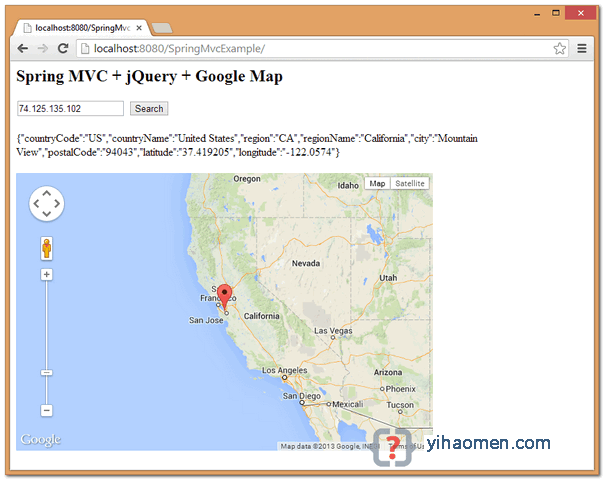
IP Address : 183.81.166.110
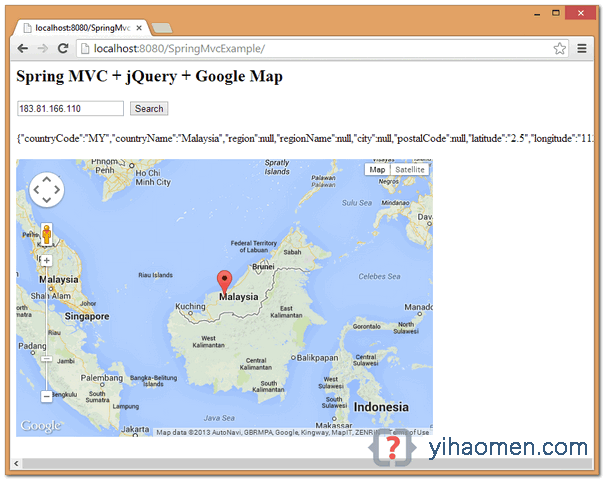
References
- Wikipedia : Geolocation software
- GeoLite Free Downloadable Databases
- Java – Find location using IP address
- Google Map APIs
- jQuery.getJSON
From:一号门
COMMENTS